 Java, Spring and Web Development tutorials 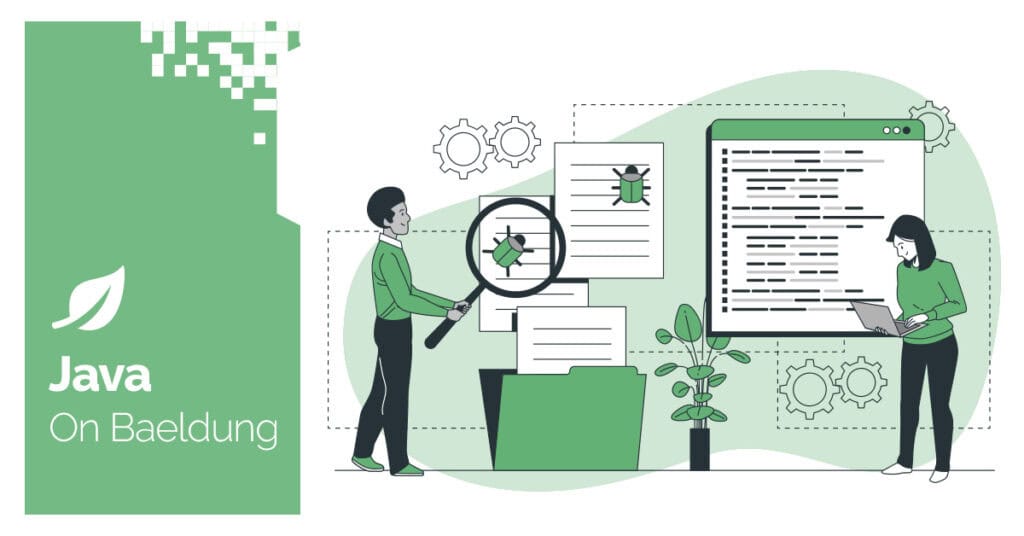 1. Overview
Apache Kogito is an open-source platform for building cloud-native business applications. In this tutorial, we’ll learn what we can do with it and how to build applications with Kogito integration.
2. What Is Kogito?
Unlike traditional business process management (BPM) tools that were created with monolithic architectures in mind, Kogito embraces a microservices-first and Kubernetes-native approach. It allows developers to model, execute, and monitor business processes and rules as standalone services that can scale independently.
Kogito’s architecture is heavily influenced by domain-specific languages (DSLs) and industry standards like BPMN 2.0 (Business Process Model and Notation) and DMN (Decision Model and Notation). These are not just visual representations — they become executable models, integrated directly into applications.
The name “Kogito” is derived from Latin “Cogito” (“I think”), whereas the “K” refers to Kubernetes and to the Knowledge Is Everything (KIE) open source business automation project from which Kogito originates.
3. Core Technologies Behind Kogito
Kogito is the modern evolution of two well-established projects that are also part of KIE:
- jBPM – for modeling and executing business processes (using Business Process Model and Notation / BPMN)
- Drools – a powerful rules engine (for decision logic using Drules Rule Language / DRL or Decision Model and Notation / DMN)
Kogito extends and modernizes these technologies by providing cloud-native packaging, Quarkus and Spring Boot support, DevOps readiness, and seamless integration with tools like Prometheus, Grafana, and OpenAPI.
4. Key Features
4.1. Cloud-Native and Kubernetes-First
Kogito applications are designed to run natively on Kubernetes. This means automatic scalability, containerization, and resilience are built-in. Developers can deploy services independently and scale decision-making or workflow logic as demand changes.
4.2. Event-Driven Architecture
Kogito supports event-driven design through integrations with Apache Kafka, enabling asynchronous communication between services. This is essential for reactive, decoupled systems that can respond in near real-time to business events.
4.3. Model-Driven Development
Kogito emphasizes model-driven development with visual tools and editors for BPMN and DMN. These models are not just documentation — they’re compiled into executable services.
Kogito offers plugins for VS Code, online editors like Kogito Tooling, and native support for frameworks like Quarkus and Spring Boot, helping Java developers build automation services with minimal friction.
4.5. DevOps-Ready
Thanks to features like automated code generation, containerized deployment, and GitOps compatibility, Kogito fits neatly into CI/CD pipelines. It supports tracing with OpenTelemetry, monitoring with Prometheus and Grafana, and health checks compatible with Kubernetes’ liveness and readiness probes.
5. Use Cases
Kogito shines in domains where rules-based decision making and workflow orchestration are essential. Some typical use cases include:
- Loan and insurance claim processing – automating complex rules for eligibility, approval, and routing
- Customer onboarding – managing multi-step workflows with compliance checks and approvals
- Supply chain management – triggering actions based on rules tied to inventory, logistics, and vendor behavior
- Healthcare workflows – handling patient journeys, documentation, and rule-driven treatment protocols
6. Sample
Let’s create a small sample that demonstrates how Kogito works. We want a rule-based credit decision service with the following rules:
- Loan applications need to contain the name and the age of the applicant as well as their income and existing debts.
- If the applicant is younger than 18, we reject the application due the applicant’s minor status.
- If the credit score is lower than 700, we reject the application as not being creditworthy.
- If the applicant’s existing debts are higher than 50% of the income, we reject the application as not being creditworthy.
- Otherwise, the application is approved.
For example, let’s send a list of applications to the service:
{
"applications": [
{
"id": "1fe1aED7-ca61-Eeb4-78fA-55f5E568Aa8b",
"person": {
"name": "Mr Smith",
"age": 25
},
"conditions": {
"creditScore": 400,
"income": 30000,
"debt": 0
}
},
{
"id": "b2c0a268-aa6f-44b8-b644-f466cb35504c",
"person": {
"name": "Mrs Doe",
"age": 35
},
"conditions": {
"creditScore": 700,
"income": 70000,
"debt": 3000
}
}
]
}
And let’s check the result:
[
{
"id": "1fe1aed7-ca61-eeb4-78fa-55f5e568aa8b",
"decision": "rejected-not-creditworthy"
},
{
"id": "b2c0a268-aa6f-44b8-b644-f466cb35504c",
"decision": "approved"
}
]
6.1. Drools Rules
We can use Drools with its Rule Language (DSL) to implement the sample. The rule file could look like:
package com.baeldung.kogito.rules
unit LoanApplicationUnit
import com.baeldung.kogito.rules.model.LoanApplication
import com.baeldung.kogito.rules.model.Person
import com.baeldung.kogito.rules.model.Conditions
import com.baeldung.kogito.rules.model.Decision
rule "only-adults"
salience 10
when
$application: /applications[
person.age < 18
]
then
modify($application){
setDecision(Decision.REJECTED_UNDERAGE)
}
end
rule "Good Credit Score And High Income"
when
$application: /applications[
person.age >= 18,
conditions.creditScore >= 700,
conditions.income > 2*conditions.debt
]
then
modify($application){
setDecision(Decision.APPROVED)
}
end
rule "Low Credit Score Or High Debt"
when
$application: /applications[
person.age >= 18,
(
conditions.creditScore < 700 ||
conditions.income <= 2*conditions.debt
)
]
then
modify($application){
setDecision(Decision.REJECTED_NOT_CREDITWORTHY)
}
end
query applications
$result: /applications
end
6.2. Kogito
If we want this to run as a microservice with an HTTP/REST endpoint, we simply create a Quarkus project and add this to the pom.xml:
<project>
<dependencyManagement>
<dependencies>
<!-- ... -->
<dependency>
<groupId>org.kie.kogito</groupId>
<artifactId>kogito-bom</artifactId>
<version>${kogito.version}</version>
<type>pom</type>
<scope>import</scope>
</dependency>
</dependencies>
</dependencyManagement>
<dependencies>
<!-- ... -->
<dependency>
<groupId>io.quarkus</groupId>
<artifactId>quarkus-resteasy-jackson</artifactId>
</dependency>
<dependency>
<groupId>org.kie.kogito</groupId>
<artifactId>kogito-quarkus-rules</artifactId>
</dependency>
</dependencies>
<properties>
<kogito.version>2.44.0.Alpha</kogito.version>
</properties>
</project>
We need to be aware that:
- the given Kogito version must support Quarkus 3
- Kogito only supports the Quarkus RESTEasy extension, but not the Quarkus REST extension
Then, put the Drools rules file under src/main/resources. Kogito detects it automatically, so we can run the Quarkus application and send the request — via curl, for example:
curl -X 'POST' \
'http://localhost:8080/applications' \
-H 'accept: application/json' \
-H 'Content-Type: application/json' \
-d '{
"applications": [
{
"id": "1fe1aED7-ca61-Eeb4-78fA-55f5E568Aa8b",
"person": {
"name": "Mr Smith",
"age": 25
},
"conditions": {
"creditScore": 400,
"income": 30000,
"debt": 0
}
},
{
"id": "b2c0a268-aa6f-44b8-b644-f466cb35504c",
"person": {
"name": "Mrs Doe",
"age": 35
},
"conditions": {
"creditScore": 700,
"income": 70000,
"debt": 3000
}
}
]
}'
As we can see, it works without any Quarkus code. We could use the existing ecosystem to build and deploy the Quarkus microservice into any cloud environment.
6.3. Custom API
Kogito is a fast way to create the REST resource without the need to write any code. However, it may make sense for us to write the code for the API ourselves. This way, we can define the custom endpoint’s URL and the JSON schema for data exchange.
When sending the request, we can see that the response body contains the whole data from the request body, supplemented by the credit decision. We might have the idea to add the Jackson or JSON-B annotations to define read-only and write-only properties, but this would not be a good idea for the Drools rule model classes because the Drools engine internally JSON-serializes and -deserializes them, so this would not work.
So, we could create our custom REST resource and DTOs, use a mapper (for example, with MapStruct) to map the DTOs to the Drools rule models, and invoke a domain service that uses the Drools rules engine. Such a domain service could look like:
import org.kie.kogito.incubation.application.AppRoot;
import org.kie.kogito.incubation.common.MapDataContext;
import org.kie.kogito.incubation.rules.RuleUnitIds;
import org.kie.kogito.incubation.rules.services.RuleUnitService;
// ...
@ApplicationScoped
public class LoanApplicationService {
@Inject
AppRoot appRoot;
@Inject
RuleUnitService ruleUnitService;
public Stream<LoanApplication> evaluate(LoanApplication... applications) {
var queryId = appRoot
.get(RuleUnitIds.class)
.get(LoanApplicationUnit.class)
.queries()
.get("applications");
var ctx = MapDataContext
.of(
Map.of("applications", applications)
);
return ruleUnitService
.evaluate(queryId, ctx)
.map(
result -> result
.as(MapDataContext.class)
.get("$result", LoanApplication.class)
);
}
}
As we can see, we use a Kogito API that is still in an incubation state. But this API allows us to integrate the Drools rules engine into any Quarkus application.
7. Conclusion
In this article, we’ve learned about Kogito as an automation tool for creating cloud-native business applications. The project is currently still undergoing a lot of development, so interfaces are constantly changing. We can use the examples available on the Kogito Examples Repository as a guide.
As always, the code presented in this article is available over on GitHub. The post Business Process Automation with Kogito first appeared on Baeldung.
Content mobilized by FeedBlitz RSS Services, the premium FeedBurner alternative. |