 Java, Spring and Web Development tutorials 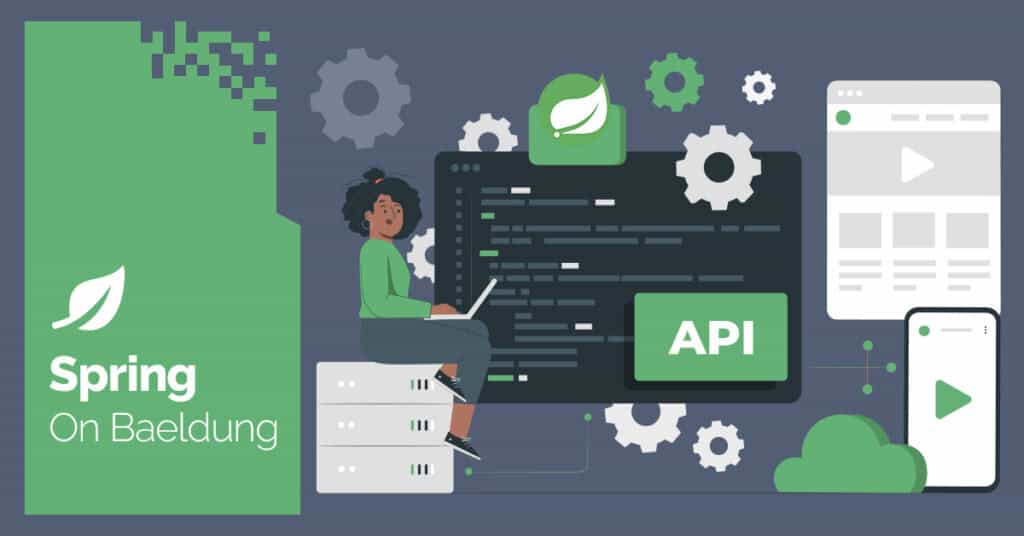 1. Introduction
When integrating MySQL with Spring Boot, we often rely on Spring Boot’s auto-configuration and JDBC support to connect to the database. However, it’s not uncommon to encounter configuration issues, especially when using outdated references. One such exception is:
Cannot load driver class: com.mysql.jdbc.Driver
In this short tutorial, we’ll understand why this error occurs, how to fix it, and test our setup to ensure the database connectivity works correctly.
2. Understanding the Cause
The root of this issue lies in using an outdated MySQL driver class. In earlier versions of the MySQL JDBC driver (Connector/J), the following driver class was commonly used:
spring.datasource.driver-class-name=com.mysql.jdbc.Driver
However, starting with MySQL Connector/J 8.0, this class has been deprecated and removed. The updated driver class is:
com.mysql.cj.jdbc.Driver
If we continue to use the old class name with a newer version of the MySQL driver, the application will fail to start with a ClassNotFoundException.
3. Fixing the Issue
To fix this problem, we need to update both our Spring Boot configuration and the dependencies being used in our project.
3.1. Using the Correct Driver Class
The first step is to replace the old driver class with the new one in the application.properties or application.yml file.
We can do it using the application.properties file:
spring.datasource.url=jdbc:mysql://localhost:3306/mydb
spring.datasource.username=root
spring.datasource.password=secret
spring.datasource.driver-class-name=com.mysql.cj.jdbc.Driver
Or we can do it using the application.yml file:
spring:
datasource:
url: jdbc:mysql://localhost:3306/mydb
username: root
password: secret
driver-class-name: com.mysql.cj.jdbc.Driver
Alternatively, Spring Boot is capable of auto-detecting the driver class from the classpath. If the correct version of the MySQL driver is present, we can safely omit the driver-class-name property altogether.
3.2. Updating Dependencies
Next, let’s ensure that the project uses the latest stable version of the MySQL JDBC driver.
For Maven:
<dependency>
<groupId>com.mysql</groupId>
<artifactId>mysql-connector-j</artifactId>
<version>9.2.0</version>
</dependency>
For Gradle:
implementation("com.mysql:mysql-connector-j:9.2.0")
Using an outdated driver version may cause incompatibilities, especially if we’re using recent versions of Spring Boot or Java.
4. Testing
After updating the configuration and dependencies, we should test the fix by verifying that the application can connect to the database.
We can write a simple integration test to ensure the DataSource is working as expected:
@SpringBootTest
class LoadDriverLiveTest {
@Autowired
private DataSource dataSource;
@Test
void whenConnectingToDatabase_thenConnectionShouldBeValid() throws Exception {
try (Connection connection = dataSource.getConnection()) {
assertNotNull(connection);
}
}
}
If the test passes, it confirms that Spring Boot has successfully loaded the driver class and established a connection to the MySQL database.
5. Conclusion
In this article, we examined the cause of the Cannot load driver class: com.mysql.jdbc.Driver exception in Spring Boot and demonstrated how to fix it by updating the driver class name and project dependencies. We also wrote a simple test to confirm that the fix works as intended.
Ensuring compatibility between your dependencies and configuration is key when working with JDBC in Spring Boot. To understand the details on this topic, we can always refer to the official Spring Boot documentation and MySQL Connector/J release notes.
As always, the implementation’s source code is available over on GitHub. The post How to Fix Exception: Cannot Load Driver Class: com.mysql.jdbc.driver in Spring Boot first appeared on Baeldung.
Content mobilized by FeedBlitz RSS Services, the premium FeedBurner alternative. |